[Cucumber] Run with Cucumber Runner
Description
Execute a feature file with a set of options using Cucumber Runner.
Keyword name: runWithCucumberRunner
Keyword syntax: runWithCucumberRunner(cucumberRunnerClass, flowControl)
Parameters
Parameter | Parameter Type | Required | Description |
---|---|---|---|
cucumberRunnerClass | Class | Yes | A class that is annotated with Cucumber runner. |
flowControl | FailureHandling | Optional | Controls the execution flow if the step fails. Specify failure handling schema to determine whether the execution should be allowed to continue or stop. |
Returns
An instance of CucumberRunnerResult
that includes status of keyword and JUnit Runner result.
Examples
Create a package called mySamplePackage under "Include/scripts/groovy" folder, then add a step definition file called myCucumberRunner within the newly created package.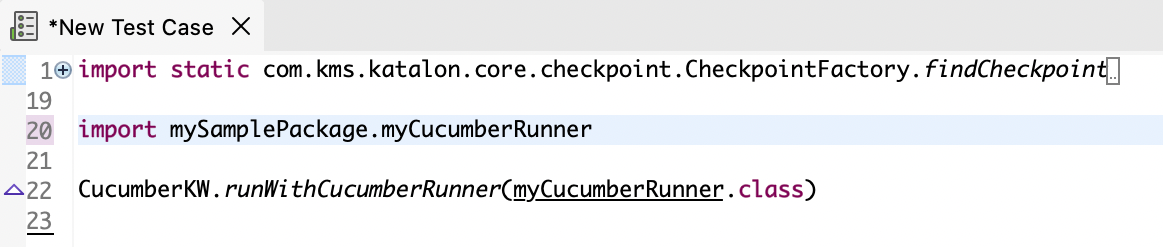
import mySamplePackage.myCucumberRunner
CucumberKW.runWithCucumberRunner(myCucumberRunner.class)
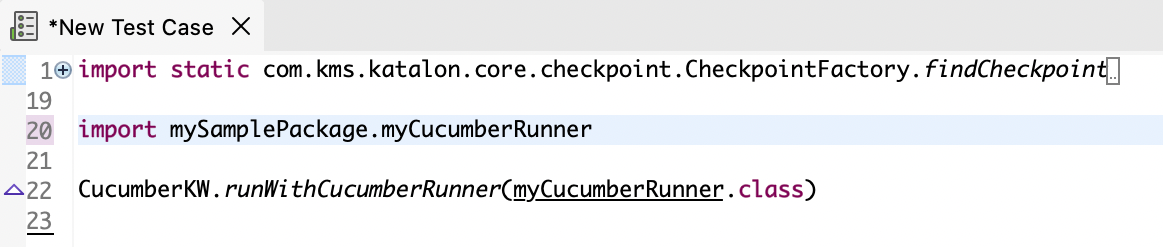
Note:
- Make sure you are not adding step definitions to the (default package).
Example 1: Run all feature files in Include/features folder. Copy and paste the code to the step definition file.
import org.junit.runner.RunWith;
import cucumber.api.CucumberOptions;
import cucumber.api.junit.Cucumber;
@RunWith(Cucumber.class)
@CucumberOptions(features = "Include/features", glue = "mySamplePackage")
public class myCucumberRunner {}
Example 2: Run all feature files in a specified file/folder. Copy and paste the code to the step definition file.
import org.junit.runner.RunWith;
import cucumber.api.CucumberOptions;
import cucumber.api.junit.Cucumber;
@RunWith(Cucumber.class)
@CucumberOptions(features = "Include/features", glue = "mySamplePackage")
public class myCucumberRunner {}
Example 3: Run all feature files in a specified file/folder, generate a JUnit Cucumber report in XML pretty format, then copy to a specified folder. Copy and paste the code to the step definition file.
import org.junit.runner.RunWith;
import cucumber.api.CucumberOptions;
import cucumber.api.junit.Cucumber;
@RunWith(Cucumber.class)
@CucumberOptions(features="Your_Folder_Path", glue="mySamplePackage", plugin = ["pretty",
"junit:Folder_Name/cucumber.xml"])
public class myCucumberRunner {}
Example 4: Run all feature files in a specified file/folder, generate multiple Cucumber reports in XML, JSON, HTML pretty format, then copy to a specified folder. Copy and paste the code to the step definition file.
import org.junit.runner.RunWith;
import cucumber.api.CucumberOptions;
import cucumber.api.junit.Cucumber;
@RunWith(Cucumber.class)
@CucumberOptions(features="Your_Folder_Path", glue="mySamplePackage", plugin = ["pretty",
"junit:Folder_Name/cucumber.xml",
"html:Folder_Name",
"json:Folder_Name/cucumber.json"])
public class myCucumberRunner {}